JavaScript for Python developers
JavaScript is similar to Python in many ways but there are some subtle differences one should definitely be aware of when first learning the language. Presented here are some of the most important and counter intuitive differences when approaching JavaScript from a Python perspective.
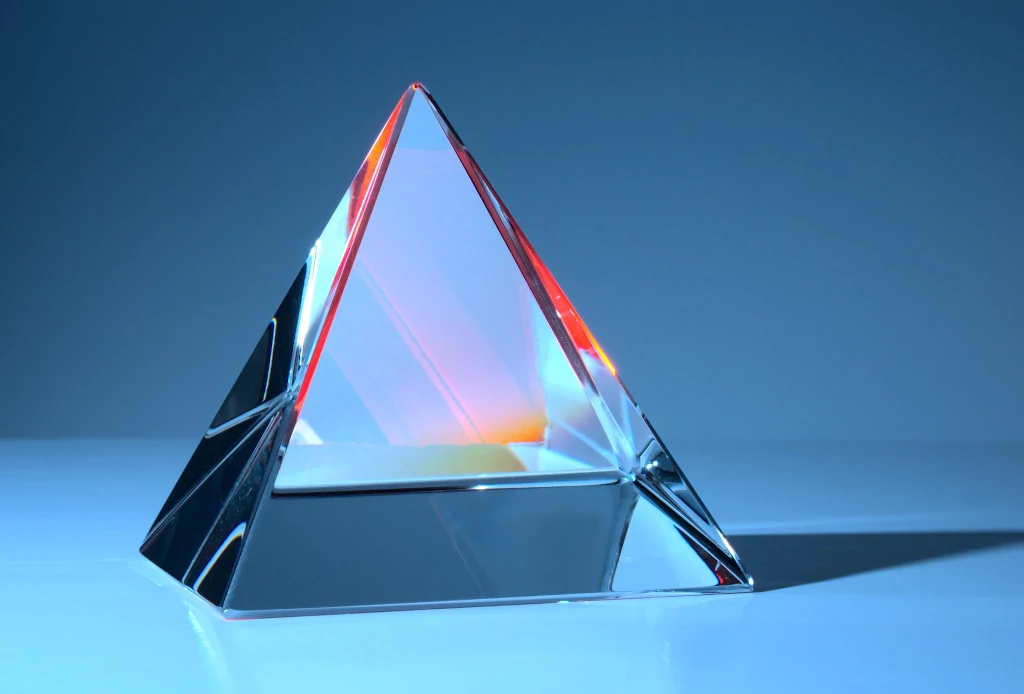
Table of Contents
- Introduction
- Truthy differences
- "In" keyword differences
- Nullish coalescing to test for null and undefined
- Array copy using spread syntax
- Negative array indices
- List and Array differences
- Python map with lambda equivalent
- Integer range
- Conclusion
- References
Introduction
JavaScript is one of the most ubiquitous languages in the world today [1, 2]. It is useful for not only front-end development but also for back-end functionality and therefore definitely a language almost all developers should know. If you are a Python developer learning JavaScript for the first time here are some tips and notable differences to help get up to speed and also avoid some potentially head-scratching bugs.
Truthy differences
One of the most important differences between Python and JavaScript is related to how values are evaluated using truthy and falsy syntax. For instance, in Python empty dictionaries and lists evaluate to a False boolean value whereas in JavaScript empty objects evaluate to a True value [3, 4]. In the example below take note how the nearly identical sets of code produce different results:
The Python code
The JavaScript code
Coming for Python perspective it is counter-intuitive for an empty JavaScript object to evaluate to a True value. This could potentially lead to some very unexpected bugs for those coming from a Python background going by assumption alone. Truthy evaluation, in JavaScript therefore, cannot be used to determine if an object is empty or not. The length property should be used instead as in the example below [5]:
The equivalent JavaScript code
"In" keyword differences
In Python one is able to use the ‘in’ keyword to determine if an element is present in a list. In JavaScript, however, the same keyword will produce a different result compared to Python. See the example below:
The Python code
The JavaScript code
The in keyword from the JavaScript code does not produce the same result as the Python equivalent. This is because the ‘in’ keyword in JavaScript cannot be used to determine if the value is present or not. It can only be used to determine if the index is present [6]. In JavaScript one should use the find() method to determine if a value is present in an array object as in this example [7]:
The equivalent JavaScript code
Nullish coalescing to test for null and undefined
In Python one can force a default when a value is None by using the ‘is’ operator and combining it with the ternary operator as in this example:
The Python code
A default value will be returned when the value being tested is None. In JavaScript one might be tempted to try and mimic the same behaviour using the or (||) operator:
The JavaScript code
The problem, however, is the right-hand side will be returned for any falsy left side value and not just for null or undefined. A more equivalent way of replicating the same Python behaviour would be to use the nullish coalescing operator “??” [8]. This operator returns the right-hand side value only when the left side is null or undefined which is more in line with the Python example above. Here’s an example of this in JavaScript:
The equivalent JavaScript code
Array copy using spread syntax
In Python there are several ways to make a copy of a list. The one I use most often is the slicing colon operator (:). The equivalent in JavaScript is the spread operator (…) [9]. Here is an example demonstrating the functionality in both languages:
The Python code
The equivalent JavaScript code
Negative array indices
In Python it is possible to deference a list using a negative index. This is when we want to retrieve a value from a list using an index number counted downward from the right-hand side of the list. Here is an example:
The Python code
In JavaScript, at first, it would seem it doesn’t provide the the same functionality for an array. However, by accessing the array with the at() method instead of brackets it is possible as seen in this example:
The equivalent JavaScript code
List and Array differences
In Python one of the most useful Abstract Data Types (ADTs) is the list [10]. JavaScript provides the same ADT in the form of an Array but with methods which are sometimes named slightly differently to the Python equivalent [11]. Here is a table with the most notable differences and similarities:
Operation | Python | JavaScript |
---|---|---|
At item to end of list/array | append(item) | push(item) |
Remove item from end of list/array | pop() | pop() |
Add item at beginning of list/array | insert(item, 0) | unshift(item) |
Remove item from the beginning of list/array | pop(0) | shift() |
Sort list/array | sort() | sort() |
Reverse items in list/array | reverse() | reverse() |
Python map with lambda equivalent
In Python we can apply a function to an iterable by using the map function. We can, furthermore, combine this functionality with a lambda anonymous function to produce a list mapping consisting of just one line of code, as in this example:
The Python code
In JavaScript we have the same functionality available by combining the Array map method and an anonymous arrow function [12]. Here’s an example:
The equivalent JavaScript code
Integer range
In Python we can combine the range function with the list function to generate a list of in sequence integer numbers, as in this example:
The Python code
In JavaScript we can recreate the same functionality by combining the Array function with the keys function as in this example:
The equivalent JavaScript code
Conclusion
Python and JavaScript are similar in many ways but there are subtle differences as shown in the examples above. Coming from a Python background one might assume the functionality is the same and then introduce bugs by using the same syntax or be unaware that the same functionality exists but in a different form. The above examples hopefully made it clear which differences are important and how to reproduce the same Python functionality in JavaScript.
References
[1] https://bootcamp.berkeley.edu/blog/most-in-demand-programming-languages/
[2] https://www.geeksforgeeks.org/top-10-programming-languages-to-learn-in-2022/
[3] https://www.freecodecamp.org/news/truthy-and-falsy-values-in-python/#-falsy-values
[4] https://developer.mozilla.org/en-US/docs/Glossary/Truthy
[5] https://stackoverflow.com/questions/679915/how-do-i-test-for-an-empty-javascript-object
[6] https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/in#basic_usage
[7] https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/find
[10] https://docs.python.org/3/tutorial/datastructures.html
[11] https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array
[12] https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/map